How to use JavaScript’s Array reduce() method?
The reduce() method in JavaScript executes a reducer function for each array element, returning a single accumulated value.
When to use the reduce() method
We should use reduce() method when we want to have a single value returned from iterating over our array.
For example:
1. Determine sum of all array element
2. Grouping similar items together
3. Removing duplicate values from an array
How to use reduce() method
let arr = [ 16, 76, 125 ];
const sum = arr.reduce( ( total, num ) => {
return total += num;
}, 0 );
console.log( sum );
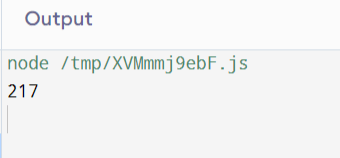