How to filter a list in dart?
In Dart, we can filter a list using the where
method, which returns an iterable containing only the elements that satisfy a given condition. Here’s how we can do it:
Example:
void main() {
List<int> numbers = [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ];
List<int> evenNumbers = numbers.where( ( number ) => number.isEven ).toList( );
print( evenNumbers );
}
Output:
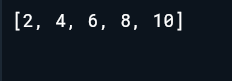
Explanation:
where( ( element ) => condition )
checks each element in the list.- It keeps only the elements that satisfy the condition.
.toList()
converts the filtered iterable into a list.