How to use push(), pop() methods of Navigator class in flutter?
In flutter we navigate from one page to another page by using Navigator class. The Navigator widget maintains a stack of roots. When we use Navigator.push()
then it adds a route at the top of the stack and in case of Navigator.pop()
it remove the route present at the top of the stack.
Let’s see the code for navigating to a page using Naviagtor.push().
Page Name: test_app.dart
import 'package:flutter/material.dart';
import 'package:flutter_first_test_project/screens/welcome_page.dart';
class TestApp extends StatelessWidget {
const TestApp({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(
"Test App",
style: TextStyle(
color: Colors.white,
),
),
),
body: Center(
child: ElevatedButton(
onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (BuildContext context)=>WelcomePage()));
},
style: ElevatedButton.styleFrom(
backgroundColor: Colors.green,
),
child: Text(
"Click",
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
),
),
);
}
}
Output:
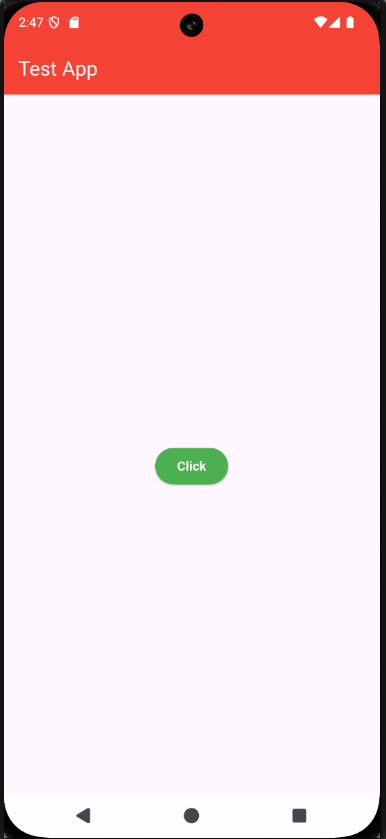
Explanation :Navigator.push(context, MaterialPageRoute(builder: (BuildContext context)=>WelcomePage()))
Using this line of code we are pushing the route of welcome_page.dart (Will show the code of this page below.) at the top of stack of routes.
Let’s see the code for navigating to a page using Naviagtor.push().
Page Name: welcome_page.dart
import 'package:flutter/material.dart';
class WelcomePage extends StatelessWidget {
const WelcomePage({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
leading: BackButton(
onPressed: () {
Navigator.pop(context);
},
),
),
body: Align(
alignment: Alignment.topCenter,
child: Text(
"Welcome to this Page",
style: TextStyle(
fontSize: 20.0,
fontWeight: FontWeight.bold,
),
),
)
),
);
}
}
Output:
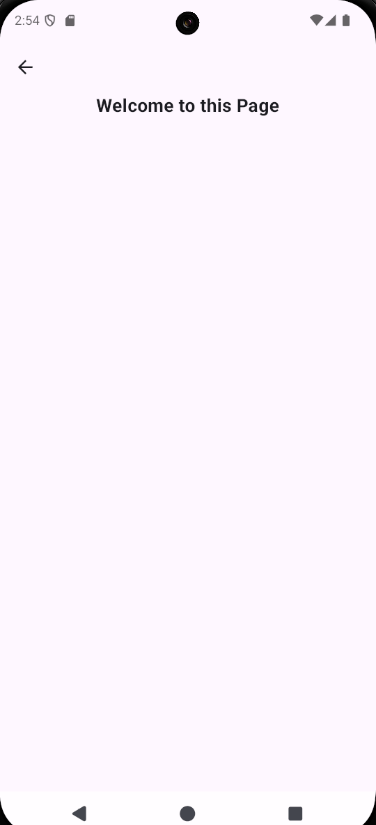
Explanation: Navigator.pop(context)
Using this line of code we are removing the route of welcome_page.dart from the top of stack of routes.
Check the small demo video of the pages (How these pages are working).