What is the use of metadata in Dart?
Metadata in Dart is used to provide additional information about code elements like classes, methods, and variables. It is typically defined using annotations, which are prefixed with the @
symbol.
Common Built-in Annotations:
Dart provides some built-in annotations:
@override
→ Overrides a method from a superclass.@deprecated
→ Marks code as deprecated.@pragma
→ Provides compiler hints.
Example:
class Parent{
void greet( ){
print( "Hello from Parent!" );
}
}
class Child extends Parent{
@override
void greet( ){
print( "Hello from Child!" );
}
}
@deprecated
void oldMethod( ){
print( "This method is deprecated." );
}
void main( ){
Child c = Child( );
c.greet( );
oldMethod( );
}
Output:
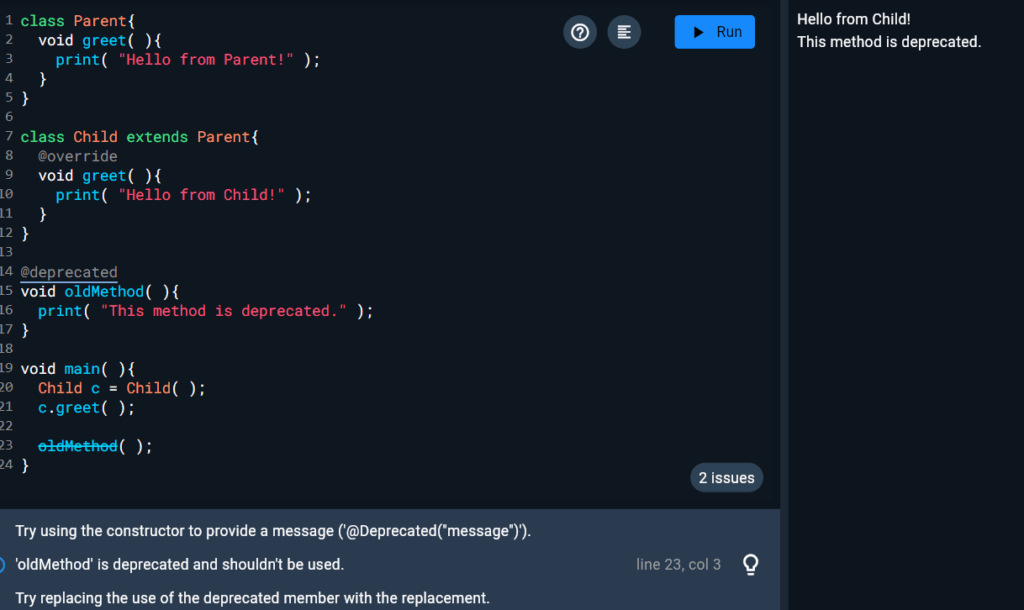