How to write a CASE statement in the SQL query?
The CASE statement functions similarly to an if/else statement in programming. It uses WHEN and THEN clauses to define specific conditions. You can specify multiple conditions, and as soon as one condition is met, the statement will stop evaluating and return the corresponding result. If none of the conditions are met, the statement will return the value specified in the ELSE clause.
If there is no ELSE clause and no conditions are true, the statement returns NULL.
--CASE Syntax--
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
WHEN conditionN THEN resultN
ELSE result
END;
--SQL CASE Examples--
SELECT user_name,
CASE
WHEN age BETWEEN 0 AND 9 THEN 'age group between 0 to 9'
WHEN age BETWEEN 10 AND 19 THEN 'age group between 10 to 19'
WHEN age BETWEEN 20 AND 29 THEN 'age group between 20 to 29'
WHEN age BETWEEN 30 AND 39 THEN 'age group between 30 to 39'
WHEN age BETWEEN 40 AND 49 THEN 'age group between 40 to 49'
WHEN age BETWEEN 50 AND 59 THEN 'age group between 50 to 59'
WHEN age BETWEEN 60 AND 69 THEN 'age group between 60 to 69'
WHEN age BETWEEN 70 AND 79 THEN 'age group between 70 to 79'
WHEN age BETWEEN 80 AND 89 THEN 'age group between 80 to 89'
WHEN age BETWEEN 90 AND 99 THEN 'age group between 90 to 99'
ELSE 'age not define'
END AS 'AgeGroup'
FROM users;
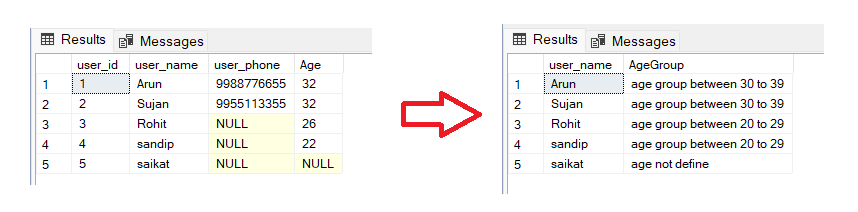