What is the use of useParams hook in React.js?
The useParams hook is used to retrieve the route parameters form the current URL. It returns an object of key/value pairs of the dynamic params from the current URL.
Example:
import React from "react";
import {
BrowserRouter as Router,
Routes,
Route,
useParams,
} from "react-router-dom";
function ProductDetails( ){
let { id } = useParams( );
return (
<div>
Product id - {id}
</div>
);
}
function Home( ){
return <h3>home page </h3>;
}
function App( ){
return (
<Router>
<Routes>
<Route path="/product/:id" element={<ProductDetails/>}/>
<Route path="/" element={<Home />}/>
</Routes>
</Router>
);
}
export default App;
OutPut:
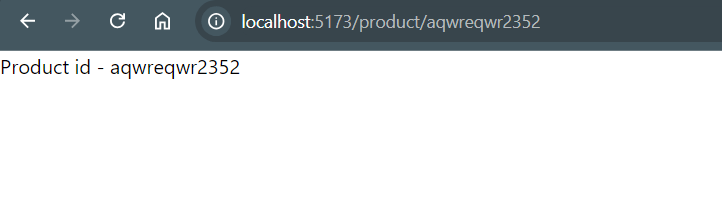